In this blog post I am going to share with you how to use UITableViewRowAction to add two table view action buttons(Delete and Share) to your table view.
Most of the code I have already available on my Swift Code Examples page and in this video I will make use of a ready to use Swift code examples to quickly put together a TableView with two action buttons, will share with you how to handle action buttons events, how to display ActionSheet and how to dismiss table view row action buttons when needed. In details the new video tutorial will cover:
- Implement UITableView programmatically
- User UITableViewDataSource and UITableViewDelegate protocols to load data into table view and make table row cells interactive
- Load three items from an array into the table view
- Determine device screen size and make table view stretch full width and height
- Implement editActionsForRowAtIndexPath to make table view cell slide to the left side and reveal action buttons
- User UITableViewRowAction to create two table view row action buttons
- Handle events when user taps on UITableViewRowAction button
- Change background color of UITableViewRowAction
- Use UIAlertController to display an Action Sheet when user taps on share action button
- Handle events when user taps on UIAlertController action buttons
Here is how our final example will look like when we are done:
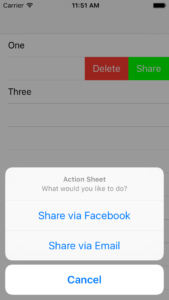
UITableViewRowAction Example in Swift – Video Tutorial
UITableViewRowAction. Add Table View Row Action Buttons to UITableView
func tableView(tableView: UITableView, editActionsForRowAtIndexPath indexPath: NSIndexPath) -> [UITableViewRowAction]? { let shareAction = UITableViewRowAction(style: .Normal, title: "Share") { (rowAction, indexPath) in print("Share Button tapped. Row item value = \(self.itemsToLoad[indexPath.row])") self.displayShareSheet(indexPath) } let deleteAction = UITableViewRowAction(style: .Default, title: "Delete") { (rowAction, indexPath) in print("Delete Button tapped. Row item value = \(self.itemsToLoad[indexPath.row])") } shareAction.backgroundColor = UIColor.greenColor() return [shareAction,deleteAction] }
UITableViewRowAction. Complete Example in Swift
import UIKit class ViewController: UIViewController, UITableViewDataSource, UITableViewDelegate { var myTableView: UITableView = UITableView() var itemsToLoad: [String] = ["One", "Two", "Three"] override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view, typically from a nib. } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() // Dispose of any resources that can be recreated. } override func viewWillAppear(animated: Bool) { super.viewWillAppear(animated) // Get main screen bounds let screenSize: CGRect = UIScreen.mainScreen().bounds let screenWidth = screenSize.width let screenHeight = screenSize.height myTableView.frame = CGRectMake(0, 0, screenWidth, screenHeight); myTableView.dataSource = self myTableView.delegate = self myTableView.registerClass(UITableViewCell.self, forCellReuseIdentifier: "myCell") self.view.addSubview(myTableView) } func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return itemsToLoad.count } func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell { let cell:UITableViewCell = tableView.dequeueReusableCellWithIdentifier("myCell", forIndexPath: indexPath) cell.textLabel?.text = self.itemsToLoad[indexPath.row] return cell } func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) { print("User selected table row \(indexPath.row) and item \(itemsToLoad[indexPath.row])") } func tableView(tableView: UITableView, editActionsForRowAtIndexPath indexPath: NSIndexPath) -> [UITableViewRowAction]? { let shareAction = UITableViewRowAction(style: .Normal, title: "Share") { (rowAction, indexPath) in print("Share Button tapped. Row item value = \(self.itemsToLoad[indexPath.row])") self.displayShareSheet(indexPath) } let deleteAction = UITableViewRowAction(style: .Default, title: "Delete") { (rowAction, indexPath) in print("Delete Button tapped. Row item value = \(self.itemsToLoad[indexPath.row])") } shareAction.backgroundColor = UIColor.greenColor() return [shareAction,deleteAction] } func displayShareSheet(indexPath: NSIndexPath) { let alertController = UIAlertController(title: "Action Sheet", message: "What would you like to do?", preferredStyle: .ActionSheet) let shareViaFacebook = UIAlertAction(title: "Share via Facebook", style: .Default, handler: { (action) -> Void in print("Send now button tapped for value \(self.itemsToLoad[indexPath.row])") }) let shareViaEmail = UIAlertAction(title: "Share via Email", style: .Default, handler: { (action) -> Void in print("Delete button tapped for value \(self.itemsToLoad[indexPath.row])") }) let cancelButton = UIAlertAction(title: "Cancel", style: .Cancel, handler: { (action) -> Void in print("Cancel button tapped") self.myTableView.setEditing(false, animated: true) }) alertController.addAction(shareViaFacebook) alertController.addAction(shareViaEmail) alertController.addAction(cancelButton) self.navigationController!.presentViewController(alertController, animated: true, completion: nil) } }
Check out these short video tutorials on how to use UITableView in Swift:
UITableView in Swift. Video Tutorials.
- Display Large Collection of Images in UITableView with SDWebImage
- UITableViewCell Separator. Hide Separator or Change Left Side Spacing
- UISegmentedControl with UITableView example in Swift. Part 1.
- UISegmentedControl with UITableView example in Swift. Part 2.